As developers, we often stick to our favorite built-in Python functions and sometimes even write custom solutions for problems that already have efficient built-in solutions. However, Python’s standard library is filled with hidden gems that can simplify our code and improve performance.
In this article, we’ll explore some lesser-known but incredibly useful Python functions that deserve more attention.
1. bisect – Efficient Sorted List Operations
The bisect
module helps in maintaining sorted lists and finding insertion points efficiently, which is especially useful when you need quick lookups or dynamic sorted sequences.
Example: Grade Tracking System
Let’s implement a system that maintains sorted grades, finds insertion points, and determines letter grades dynamically.
from bisect import bisect_left, bisect_right, insort grades = [60, 70, 75, 85, 90, 95] # Find the correct position to insert a new grade new_grade = 82 position = bisect_left(grades, new_grade) print(f"Insert 82 at position: {position}") # Insert while keeping the list sorted insort(grades, new_grade) print(f"Grades after insertion: {grades}") # Assign letter grades based on score def grade_to_letter(score): breakpoints = [60, 70, 80, 90] # F, D, C, B, A grades = 'FDCBA' position = bisect_right(breakpoints, score) return grades[position] print(f"Score 82 gets grade: {grade_to_letter(82)}") print(f"Score 75 gets grade: {grade_to_letter(75)}")

2. itertools.pairwise – Process Consecutive Pairs Easily
The pairwise
function generates consecutive pairs in a sequence, making it great for trend analysis, computing differences, and smoothing data.
Example: Temperature Change Analysis
When analyzing temperature data, we often need to calculate differences between consecutive readings, find trends, or compute moving averages. Instead of manually iterating with index-based logic, the pairwise
function from itertools allows us to process consecutive pairs effortlessly.
from itertools import pairwise temperatures = [20, 23, 24, 25, 23, 22, 20] # Compute temperature differences changes = [curr - prev for prev, curr in pairwise(temperatures)] print("Temperature changes:", changes) # Compute moving averages moving_averages = [(t1 + t2) / 2 for t1, t2 in pairwise(temperatures)] print("Moving averages:", moving_averages) # Find the largest temperature jump max_jump = max(abs(b - a) for a, b in pairwise(temperatures)) print(f"Largest temperature change: {max_jump} degrees")

3. statistics.fmean – Fast and Precise Floating-Point Mean
The fmean
function computes the mean faster and with higher precision compared to mean()
, making it great for large datasets.
Example: Speed Comparison of mean() vs fmean()
The example demonstrates a performance comparison between two functions from Python’s statistics module: mean()
and fmean()
.
from statistics import mean, fmean import time temperatures = [21.5, 22.1, 23.4, 22.8, 21.8] * 100000 # Regular mean start_time = time.perf_counter() regular_mean = mean(temperatures) regular_time = time.perf_counter() - start_time # fmean start_time = time.perf_counter() fast_mean = fmean(temperatures) fast_time = time.perf_counter() - start_time print(f"Regular mean: {regular_mean:.10f} (took {regular_time:.4f} seconds)") print(f"fmean: {fast_mean:.10f} (took {fast_time:.4f} seconds)")

fmean
is significantly faster, especially for large datasets.
4. itertools.takewhile – Process Until a Condition Fails
The takewhile
function helps process sequences until a condition is no longer met, offering a cleaner way to break loops.
Example: Processing Logs Until an Error Occurs
This example demonstrates how to process log entries efficiently until an error is encountered using Python’s itertools.takewhile
function. The goal is to extract and display all log entries that occur before the first error entry in a list of logs.
from itertools import takewhile log_entries = [ "INFO: System started", "INFO: Loading data", "INFO: Processing users", "ERROR: Database connection failed", "INFO: Retrying connection", ] # Retrieve logs until the first error normal_operation = list(takewhile(lambda x: not x.startswith("ERROR"), log_entries)) print("Logs before first error:") print("n".join(normal_operation))

5. operator.attrgetter – Efficiently Retrieve Object Attributes
The attrgetter
function simplifies extracting attributes from objects, especially for sorting and nested attributes.
Example: Sorting Blog Articles by Author and Views
This code demonstrates how to sort a list of blog articles based on multiple criteria using Python’s sorted()
function with the attrgetter utility from the operator module.
The sorting is performed first by the author’s name (alphabetically) and then by the number of views (numerically). This approach is useful when you want to organize data hierarchically, such as grouping articles by author and ranking them by popularity.
from operator import attrgetter from datetime import datetime class Article: def __init__(self, title, author, views, date): self.title = title self.author = author self.stats = type('Stats', (), {'views': views}) self.date = date def __repr__(self): return f"{self.title} by {self.author}" articles = [ Article("Python Tips", "Alice", 1500, datetime(2025, 1, 15)), Article("Data Science", "Bob", 2500, datetime(2025, 1, 20)), Article("Web Dev", "Alice", 1800, datetime(2025, 1, 10)) ] # Sort by author and then by views sorted_articles = sorted(articles, key=attrgetter('author', 'stats.views')) for article in sorted_articles: print(f"{article.author}: {article.title} ({article.stats.views} views)")
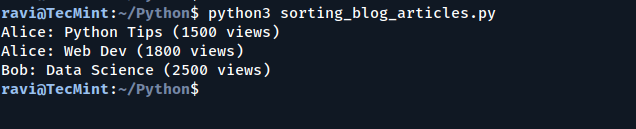
6. itertools.chain.from_iterable – Flatten Nested Lists Efficiently
The itertools.chain.from_iterable
function is a powerful tool in Python’s itertools module that allows you to flatten nested iterable structures (e.g., lists of lists, tuples of tuples, etc.) into a single iterable in a memory-efficient manner.
Unlike other methods of flattening, such as list comprehensions or nested loops, chain.from_iterable
avoids creating intermediate lists, making it particularly useful for handling large datasets.
Example: Flattening Sales Data
This example demonstrates how to efficiently flatten a nested list structure using Python’s itertools.chain
. The scenario involves sales data represented as a list of sublists, where each sublist contains tuples representing monthly sales figures.
The goal is to “flatten” this nested structure into a single list of tuples for easier processing or analysis.
from itertools import chain sales_data = [ [('Jan', 100), ('Feb', 150)], [('Mar', 200), ('Apr', 180)], [('May', 210), ('Jun', 190)] ] # Flatten data efficiently flat_sales = list(chain.from_iterable(sales_data)) print("Flattened sales data:", flat_sales)

7. functools.cache – Automatic Function Caching
The cache
decorator (introduced in Python 3.9) stores function results to speed up repeated computations, ideal for recursive or expensive calculations.
Example: Fibonacci Sequence Optimization
This example demonstrates how `functools.cache
` significantly improves performance by storing previously computed results. Instead of recalculating values for each recursive call, it retrieves cached results, making functions like Fibonacci calculations exponentially faster.
from functools import cache @cache def fibonacci(n): if n < 2: return n return fibonacci(n - 1) + fibonacci(n - 2) print(fibonacci(30)) # Much faster than an uncached version
Output:
832040
8. contextlib.suppress – Cleaner Exception Handling
The suppress
function is a cleaner alternative to try-except when you want to ignore specific exceptions without unnecessary boilerplate code.
Example: Suppressing File Not Found Errors
This example attempts to open a file that may not exist. Instead of raising a FileNotFoundError, the suppress()
function silently handles it, allowing the program to continue without interruption.
from contextlib import suppress with suppress(FileNotFoundError): with open("non_existent_file.txt", "r") as file: data = file.read() print("No crash, program continues!")
Output:
No crash, program continues!
9. pathlib.Path.glob – Intuitive File Searching
The glob()
method from pathlib.Path
allows you to search for files that match a specific pattern within a directory. Using rglob()
enables recursive searching, meaning it looks through all subdirectories as well, which is useful for tasks like finding all Python scripts in a project folder without manually checking each directory.
Example: Finding All Python Files Recursively
This example uses Path.rglob("*.py")
to recursively search for all Python files (.py)
in the current directory and its subdirectories. The result is a list of file paths, making it easy to manage or process multiple Python scripts at once.
from pathlib import Path python_files = list(Path(".").rglob("*.py")) print("Found Python files:", python_files)

10. dataclasses.asdict – Convert Objects to Dictionaries Easily
The asdict()
function from dataclasses simplifies converting objects into dictionaries, making them JSON-serializable or easy to manipulate.
Example: Converting a User Object to JSON
Transforms a dataclass
object into a dictionary, making it easy to serialize into JSON.
from dataclasses import dataclass, asdict import json @dataclass class User: name: str age: int email: str user = User("Alice", 30, "[email protected]") user_dict = asdict(user) print(json.dumps(user_dict, indent=4)) # Easily convert to JSON
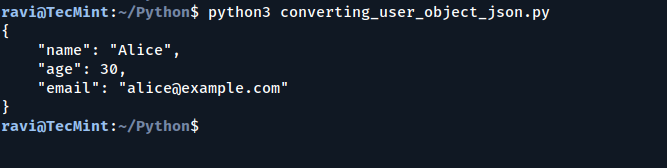
Conclusion
These lesser-known Python functions simplify complex operations while improving readability and efficiency. Try integrating them into your projects, and you’ll be surprised at how much cleaner and faster your code becomes!