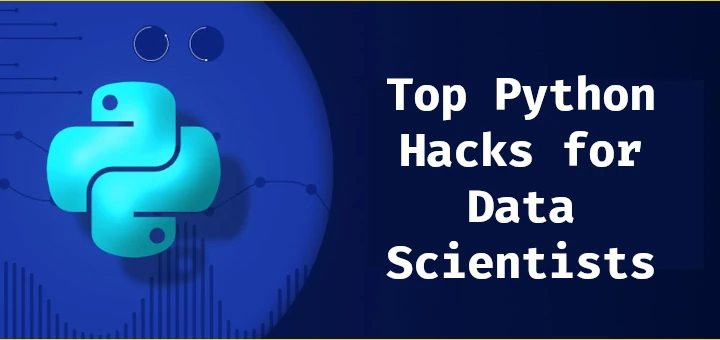
As a data scientist, you likely spend a lot of your time writing Python code, which is known for being easy to learn and incredibly versatile and it can handle almost any task you throw at it.
But even if you’re comfortable with the basics, there are some advanced tricks that can take your skills to the next level and help you write cleaner, faster, and more efficient code, saving you time and effort in your projects.
In this article, we’ll explore 10 advanced Python tricks that every data professional should know. Whether you’re simplifying repetitive tasks, optimizing your workflows, or just making your code more readable, these techniques will give you a solid edge in your data science work.
1. List Comprehensions for Concise Code
List comprehensions are a Pythonic way to create lists in a single line of code. They’re not only concise but also faster than traditional loops.
For example, instead of writing:
squares = [] for x in range(10): squares.append(x**2)
You can simplify it to:
squares = [x**2 for x in range(10)]
This trick is especially useful for data preprocessing and transformation tasks.
2. Leverage Generators for Memory Efficiency
Generators are a great way to handle large datasets without consuming too much memory. Unlike lists, which store all elements in memory, generators produce items on the fly.
For example:
def generate_numbers(n): for i in range(n): yield i
Use generators when working with large files or streaming data to keep your memory usage low.
3. Use zip to Iterate Over Multiple Lists
The zip
function allows you to iterate over multiple lists simultaneously, which is particularly handy when you need to pair related data points.
For example:
names = ["Alice", "Bob", "Charlie"] scores = [85, 90, 95] for name, score in zip(names, scores): print(f"{name}: {score}")
This trick can simplify your code when dealing with parallel datasets.
4. Master enumerate for Index Tracking
When you need both the index and the value of items in a list, use enumerate
instead of manually tracking the index.
For example:
fruits = ["apple", "banana", "cherry"] for index, fruit in enumerate(fruits): print(f"Index {index}: {fruit}")
This makes your code cleaner and more readable.
5. Simplify Data Filtering with filter
The filter
function allows you to extract elements from a list that meet a specific condition.
For example, to filter even numbers:
numbers = [1, 2, 3, 4, 5, 6] evens = list(filter(lambda x: x % 2 == 0, numbers))
This is a clean and functional way to handle data filtering.
6. Use collections.defaultdict for Cleaner Code
When working with dictionaries, defaultdict
from the collections
module can save you from checking if a key exists.
For example:
from collections import defaultdict word_count = defaultdict(int) for word in ["apple", "banana", "apple"]: word_count[word] += 1
This eliminates the need for repetitive if-else
statements.
7. Optimize Data Processing with map
The map
function applies a function to all items in an iterable.
For example, to convert a list of strings to integers:
strings = ["1", "2", "3"] numbers = list(map(int, strings))
This is a fast and efficient way to apply transformations to your data.
8. Unpacking with *args and **kwargs
Python’s unpacking operators (*args
and **kwargs
) allow you to handle variable numbers of arguments in functions.
For example:
def summarize(*args): return sum(args) print(summarize(1, 2, 3, 4)) # Output: 10
This is particularly useful for creating flexible and reusable functions.
9. Use itertools for Advanced Iterations
The itertools
module provides powerful tools for working with iterators. For example, itertools.combinations
can generate all possible combinations of a list:
import itertools letters = ['a', 'b', 'c'] combinations = list(itertools.combinations(letters, 2))
This is invaluable for tasks like feature engineering or combinatorial analysis.
10. Automate Workflows with contextlib
The contextlib
module allows you to create custom context managers, which are great for automating setup and teardown tasks.
For example:
from contextlib import contextmanager @contextmanager def open_file(file, mode): f = open(file, mode) try: yield f finally: f.close() with open_file("example.txt", "w") as f: f.write("Hello, World!")
This ensures resources are properly managed, even if an error occurs.
11. Pandas Profiling for Quick Data Exploration
Exploring datasets can be time-consuming, but pandas_profiling
makes it a breeze, as this library generates a detailed report with statistics, visualizations, and insights about your dataset in just one line of code:
import pandas as pd from pandas_profiling import ProfileReport df = pd.read_csv("your_dataset.csv") profile = ProfileReport(df, explorative=True) profile.to_file("report.html")
This trick is perfect for quickly understanding data distributions, missing values, and correlations.
12. F-Strings for Cleaner String Formatting
F-strings, introduced in Python 3.6, are a game-changer for string formatting. They’re concise, readable, and faster than older methods like %
formatting or str.format()
.
For example:
name = "Alice" age = 30 print(f"{name} is {age} years old.")
You can even embed expressions directly:
print(f"{name.upper()} will be {age + 5} years old in 5 years.")
F-strings make your code cleaner and more intuitive.
13. Lambda Functions for Quick Operations
Lambda functions are small, anonymous functions that are perfect for quick, one-off operations. They’re especially useful with functions like map()
, filter()
, or sort()
.
For example:
numbers = [1, 2, 3, 4, 5] squared = list(map(lambda x: x**2, numbers))
Lambda functions are great for simplifying code when you don’t need a full function definition.
14. NumPy Broadcasting for Efficient Computations
NumPy broadcasting allows you to perform operations on arrays of different shapes without explicitly looping.
For example:
import numpy as np array = np.array([[1, 2, 3], [4, 5, 6]]) result = array * 2 # Broadcasting multiplies every element by 2
This trick is incredibly useful for vectorized operations, making your code faster and more efficient.
15. Matplotlib Subplots for Multi-Plot Visualizations
Creating multiple plots in a single figure is easy with Matplotlib’s subplots function.
For example:
import matplotlib.pyplot as plt fig, axes = plt.subplots(2, 2) # 2x2 grid of subplots axes[0, 0].plot([1, 2, 3], [4, 5, 6]) # Plot in the first subplot axes[0, 1].scatter([1, 2, 3], [4, 5, 6]) # Scatter plot in the second subplot plt.show()
This is perfect for comparing multiple datasets or visualizing different aspects of your data side by side.
16. Scikit-learn Pipelines for Streamlined Machine Learning
Scikit-learn’s Pipeline
class helps you chain multiple data preprocessing and modeling steps into a single object, which ensures reproducibility and simplifies your workflow.
For example:
from sklearn.pipeline import Pipeline from sklearn.preprocessing import StandardScaler from sklearn.linear_model import LogisticRegression pipeline = Pipeline([ ('scaler', StandardScaler()), ('classifier', LogisticRegression()) ]) pipeline.fit(X_train, y_train)
Pipelines are a must-have for organizing and automating machine learning workflows.
Final Thoughts
These advanced Python tricks can make a big difference in your data science projects. So, the next time you’re working on a data science project, try implementing one or more of these tricks. You’ll be amazed at how much time and effort you can save!
If you’re looking to deepen your data science skills, here are some highly recommended courses that can help you master Python and data science:
- Data Science Specialization by Coursera – This comprehensive specialization by Johns Hopkins University covers everything from Python programming to machine learning and data visualization, which is perfect for beginners and intermediate learners.
- Python for Data Science and Machine Learning Bootcamp by Udemy – This best-selling course on Udemy provides hands-on experience with Python libraries like Pandas, NumPy, Matplotlib, and Scikit-learn.
- Introduction to Data Science with Python by edX – This course by Harvard University is an excellent introduction to data science using Python that covers data analysis, visualization, and machine learning.
- Data Science Career Track by DataCamp – DataCamp’s career track offers a structured learning path with interactive exercises. It covers Python, SQL, machine learning, and more, making it a great choice for aspiring data scientists.
By enrolling in these courses, you’ll gain the knowledge and skills needed to excel in data science while applying the advanced Python tricks covered in this article.
Disclaimer: Some of the links in this article are affiliate links, which means I may earn a small commission if you purchase a course through them. This comes at no extra cost to you and helps support the creation of free, high-quality content like this.
Thank you for your support!