Appending text to files is a common task in systems administration and development, especially when dealing with large datasets or logs.
This can be efficiently accomplished using a Bash script, which is a powerful command-line shell in Unix-like operating systems, offering a range of utilities and operators to manipulate files, including the ability to append text.
In this article, we will walk you through the process of appending text to a single file and then expand that to appending text to multiple files using a Bash script, which is a useful skill when you need to automate text insertion in log files, configuration files, or scripts.
Prerequisites
Before we get into the scripting part, make sure you have basic familiarity with the Bash shell and text editors (e.g., nano, vim, or emacs).
Appending Text to a Single File
The simplest method to append text to a single file in Bash is by using the echo command with the append operator (>>)
.
echo "This is the appended text" >> filename.txt
Explanation:
echo "This is the appended text"
: This command outputs the text you want to append.>> filename.txt
: The>>
operator appends the output of theecho
command to the specified file, in this case,filename.txt
.
If filename.txt
does not already exist, it will be created. If the file exists, the text will be added at the end of the file.
You can also append the output of commands to files. For instance, if you want to append the current date and time to a file, you can do:
echo "Current date and time: $(date)" >> log.txt
This will append something like:
Current date and time: Mon May 7 14:22:34 UTC 2025
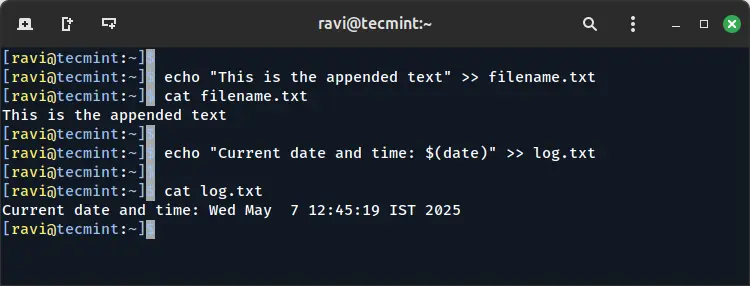
Appending Text to Multiple Files Using a Bash Script
If you need to append text to multiple files, you can use a Bash script, which is especially useful when working with directories containing many files, such as log files, configuration files, or output files generated by different processes.
Suppose you want to append the same text to all .txt
files in a specific directory, you can create a Bash script called ‘append_text.sh
‘ with your choice of editor.
vi append_text.sh OR nano append_text.sh
Next, copy and paste the following script code into a file.
#!/bin/bash # Directory containing the files DIRECTORY="/path/to/directory" # Text to append TEXT="This is the appended text for all files." # Loop through each .txt file in the directory for FILE in "$DIRECTORY"/*.txt; do if [ -f "$FILE" ]; then # Ensure it's a file echo "$TEXT" >> "$FILE" echo "Appended text to $FILE" fi done
Explanation:
DIRECTORY="/path/to/directory"
: The directory containing the files you want to modify, make sure to replace this with the actual path to your directory.TEXT="This is the appended text for all files."
: The text you want to append to each file.for FILE in "$DIRECTORY"/*.txt; do
: Thisfor
loop iterates over each.txt
file in the specified directory.if [ -f "$FILE" ]; then
: This condition ensures that the script only processes regular files (not directories or symlinks).echo "$TEXT" >> "$FILE"
: Theecho
command appends the text to the current file.echo "Appended text to $FILE"
: This prints a confirmation message for each file processed.
To execute this script, save it to a file (e.g., append_text.sh
), give it execute permissions, and run it:
chmod +x append_text.sh ./append_text.sh
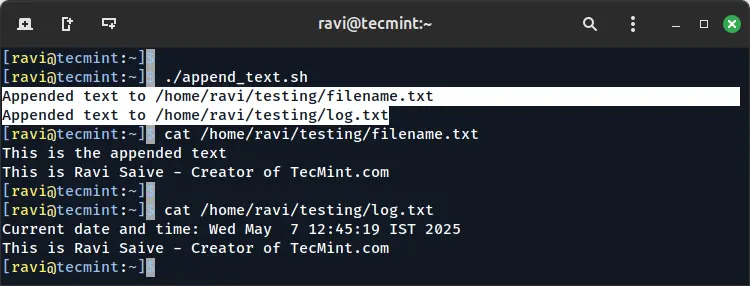
Appending Text to Files Based on a Condition
You may want to append text only if a specific condition is met, such as appending text to files that do not already contain it.
Here’s how you can modify the script to do this:
#!/bin/bash DIRECTORY="/path/to/directory" TEXT="This is the appended text for all files." for FILE in "$DIRECTORY"/*.txt; do if [ -f "$FILE" ]; then # Check if the text already exists in the file if ! grep -q "$TEXT" "$FILE"; then echo "$TEXT" >> "$FILE" echo "Appended text to $FILE" else echo "Text already exists in $FILE, skipping." fi fi done
Explanation:
if ! grep -q "$TEXT" "$FILE"; then
: Thegrep -q
command checks if the specified text already exists in the file. The!
negates the result, so if the text is not found, it proceeds to append the text.
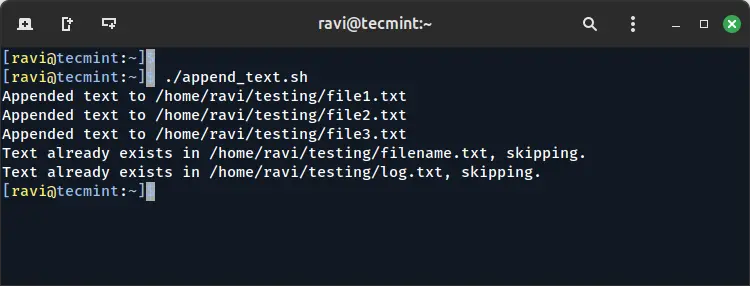
Conclusion
Appending text to files using a Bash script is a powerful and flexible technique that can be customized to suit various needs. Whether you need to append a static string to one file or modify multiple files in bulk, Bash scripts provide a simple yet effective solution.
Remember to always test your script on a small set of files to ensure it behaves as expected before running it on a large scale. Additionally, be mindful of file permissions and make backups if needed to prevent accidental data loss.